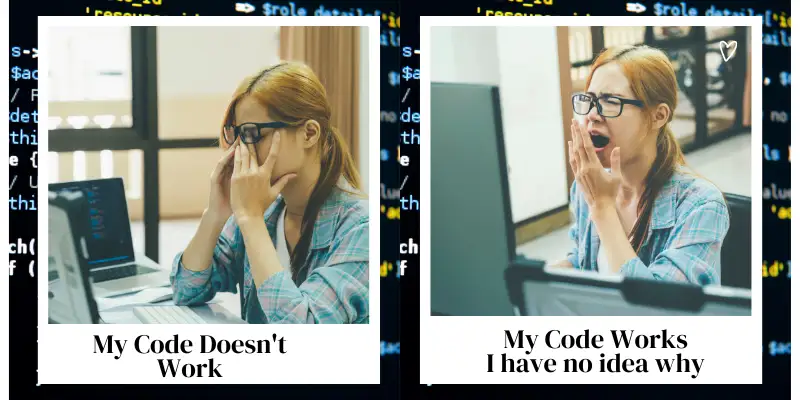
Mastering Clean Code Principles in Android Development
- softscribble@gmail.com
- May 22, 2024
- Software development
- 0 Comments
Clean code is the foundation of maintainable, scalable, and robust software. In the fast-paced world of Android app development, adhering to clean code principles ensures your codebase remains comprehensible and easy to manage, even as your application evolves. Whether you’re an individual developer or part of an Android app development company, mastering these principles is essential. Let’s dive into six fundamental concepts: MVVM, Dependency Injection (DI), Single Responsibility Principle (SRP), Test-Driven Development (TDD), Function Structure, and Class Structure.
1. Model-View-ViewModel (MVVM)
The MVVM architecture pattern is a cornerstone of clean code in Android development. It separates the user interface logic from the business logic by introducing a ViewModel layer, promoting a clear division of responsibilities.
Breaking Down MVVM
- Models: These represent your application’s data and business logic. They are responsible for managing the state and ensuring the data integrity of your application.
- Views: These are the UI components that display data to the user and capture user input. Think of Activities, Fragments, and XML layouts in Android.
- ViewModels: Acting as intermediaries between Views and Models, ViewModels expose data streams and handle user interactions. They provide a clean API for the UI to interact with the underlying data and business logic.
Why MVVM?
MVVM enhances testability and modularity. With this separation, you can test your business logic independently of the UI, making your codebase more maintainable and less prone to bugs.
2. Dependency Injection (DI)
Dependency Injection is a design pattern that improves the manageability and testability of your code by decoupling component creation from component usage.
Implementing DI in Android
In Android, popular DI frameworks like Dagger and Hilt are used to inject dependencies. These frameworks automatically provide the necessary dependencies to your classes, which:
- Improves Testability: By injecting mocks or stubs during testing, you can isolate and test specific components.
- Enhances Maintainability: Centralized dependency management makes it easier to update and manage dependencies across your project.
Example: Using Hilt for Dependency Injection
3. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change, meaning it should focus on a single responsibility or purpose. This principle promotes cleaner, more understandable, and easier-to-maintain code.
Applying SRP in Android
Breaking down functionality into smaller, focused classes or methods:
- Enhances Readability: Each class or method has a clear and singular purpose.
- Facilitates Testing: Smaller units of code are easier to test and debug.
- Promotes Reusability: Single-purpose classes and methods can often be reused across different parts of your application.
Example: Refactoring a Monolithic Class
Before applying SRP:
After applying SRP:
4. Test-Driven Development (TDD)
Test-Driven Development is a disciplined approach to software development where you write tests before writing the actual code. This ensures your codebase is always testable and that new features are well-defined and robust.
Benefits of TDD
- Defines Clear Requirements: Writing tests first forces you to think through the requirements and expected behavior of your code.
- Ensures Resilience: With a comprehensive test suite, you can confidently make changes without fear of breaking existing functionality.
- Improves Code Quality: TDD encourages simpler and more modular code, as complex code tends to be harder to test.
Example: A Simple TDD Workflow
- Write a failing test for a new feature.
- Implement the minimal code required to pass the test.
- Refactor the code while ensuring all tests still pass.
5. Function Structure
Writing clean, well-structured functions is crucial for readability and maintainability.
Best Practices for Function Structure
- Descriptive Names: Choose meaningful names that convey the purpose and behavior of the function.
- Parameter Usage: Pass inputs through parameters and use return values or side effects to produce outputs.
- Method Naming Conventions: Follow conventions like camelCase and use verb-noun combinations for clarity.
- Break Down Complexity: Split complex methods into smaller, reusable functions.
- Error Handling: Implement error handling and exception management to handle unexpected situations gracefully.
- Documentation: Write clear comments to explain the function’s purpose, parameters, return values, and side effects.
Example: Refactoring a Complex Method
Before refactoring:
After refactoring:
6. Class Structure
A well-defined class structure is essential for clean code. Properly structured classes are easier to understand, maintain, and extend.
Best Practices for Class Structure
- Identify Responsibilities: Determine the roles and responsibilities of each class based on requirements and design. Use SRP to ensure each class has a single, well-defined purpose.
- Define Class Members: Declare attributes to represent state and behavior. Encapsulate fields using access modifiers and provide getter and setter methods if needed.
- Implement Methods: Define methods to encapsulate behavior and functionality. Follow naming conventions and break down complex methods.
- Error Handling: Implement robust error handling and exception management.
- Documentation: Write clear comments or documentation for methods and classes.
Example: Structuring a Class
Conclusion
By mastering these clean code principles, you can create Android applications that are not only functional but also maintainable, scalable, and robust. Whether you’re working solo or part of an Android app development company, these practices will significantly improve your code quality and project success.
Embrace MVVM to separate concerns, utilize Dependency Injection for better management of dependencies, adhere to the Single Responsibility Principle for focused classes and methods, practice Test-Driven Development to ensure robust and testable code, and follow best practices for function and class structures. Your future self—and your team—will thank you for it!
Tags: Android App Development, Android App Development Company, android app development company usa, Code Principles in Android
Contact Softscribble for your software Requirement
Contact Now