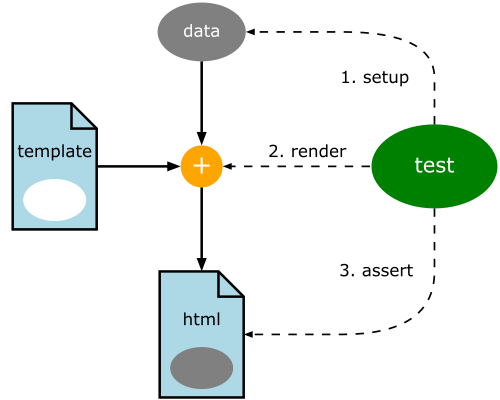
Test-Driving HTML Templates
- softscribble@gmail.com
- May 22, 2024
- Software development
- 0 Comments
After a decade or more where Single-Page-Applications generated by
JavaScript frameworks have
become the norm, we see that server-side rendered HTML is becoming
popular again, also thanks to libraries such as HTMX or Turbo. Writing a rich web UI in a
traditionally server-side language like Go or Java is now not just possible,
but a very attractive proposition.
We then face the problem of how to write automated tests for the HTML
parts of our web applications. While the JavaScript world has evolved powerful and sophisticated ways to test the UI,
ranging in size from unit-level to integration to end-to-end, in other
languages we do not have such a richness of tools available.
When writing a web application in Go or Java, HTML is commonly generated
through templates, which contain small fragments of logic. It is certainly
possible to test them indirectly through end-to-end tests, but those tests
are slow and expensive.
We can instead write unit tests that use CSS selectors to probe the
presence and correct content of specific HTML elements within a document.
Parameterizing these tests makes it easy to add new tests and to clearly
indicate what details each test is verifying. This approach works with any
language that has access to an HTML parsing library that supports CSS
selectors; examples are provided in Go and Java.
Motivation
Why test-drive HTML templates? After all, the most reliable way to check
that a template works is to render it to HTML and open it in a browser,
right?
There’s some truth in this; unit tests cannot prove that a template
works as expected when rendered in a browser, so checking them manually
is necessary. And if we make a
mistake in the logic of a template, usually the template breaks
in an obvious way, so the error is quickly spotted.
On the other hand:
- Relying on manual tests only is risky; what if we make a change that breaks
a template, and we don’t test it because we did not think it would impact the
template? We’d get an error at runtime! - Templates often contain logic, such as if-then-else’s or iterations over arrays of items,
and when the array is empty, we often need to show something different.
Manual checking all cases, for all of these bits of logic, becomes unsustainable very quickly - There are errors that are not visible in the browser. Browsers are extremely
tolerant of inconsistencies in HTML, relying on heuristics to fix our broken
HTML, but then we might get different results in different browsers, on different devices. It’s good
to check that the HTML structures we are building in our templates correspond to
what we think.
It turns out that test-driving HTML templates is easy; let’s see how to
do it in Go and Java. I will be using as a starting point the TodoMVC
template, which is a sample application used to showcase JavaScript
frameworks.
We will see techniques that can be applied to any programming language and templating technology, as long as we have
access to a suitable HTML parser.
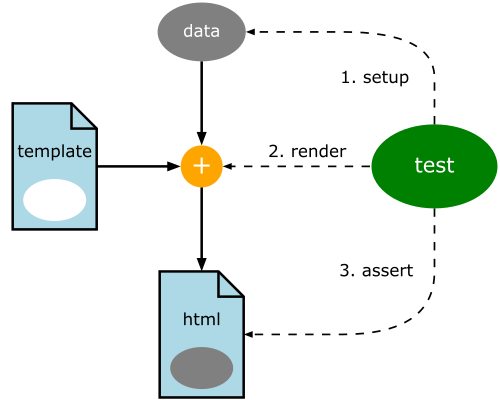
This article is a bit long; you may want to take a look at the
final solution in Go or
in Java,
or jump to the conclusions.
Level 1: checking for sound HTML
The number one thing we want to check is that the HTML we produce is
basically sound. I don’t mean to check that HTML is valid according to the
W3C; it would be cool to do it, but it’s better to start with much simpler and faster checks.
For instance, we want our tests to
break if the template generates something like
<div>foo</p>
Let’s see how to do it in stages: we start with the following test that
tries to compile the template. In Go we use the standard html/template
package.
Go
func Test_wellFormedHtml(t *testing.T) { templ := template.Must(template.ParseFiles("index.tmpl")) _ = templ }
In Java, we use jmustache
because it’s very simple to use; Freemarker or
Velocity are other common choices.
Java
@Test void indexIsSoundHtml() { var template = Mustache.compiler().compile( new InputStreamReader( getClass().getResourceAsStream("/index.tmpl"))); }
If we run this test, it will fail, because the index.tmpl
file does
not exist. So we create it, with the above broken HTML. Now the test should pass.
Then we create a model for the template to use. The application manages a todo-list, and
we can create a minimal model for demonstration purposes.
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Must(template.ParseFiles("index.tmpl"))
model := todo.NewList()
_ = templ
_ = model
}
Java
@Test
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var model = new TodoList();
}
Now we render the template, saving the results in a bytes buffer (Go) or as a String
(Java).
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Must(template.ParseFiles("index.tmpl"))
model := todo.NewList()
var buf bytes.Buffer
err := templ.Execute(&buf, model)
if err != nil {
panic(err)
}
}
Java
@Test
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var model = new TodoList();
var html = template.execute(model);
}
At this point, we want to parse the HTML and we expect to see an
error, because in our broken HTML there is a div
element that
is closed by a p
element. There is an HTML parser in the Go
standard library, but it is too lenient: if we run it on our broken HTML, we don’t get an
error. Luckily, the Go standard library also has an XML parser that can be
configured to parse HTML (thanks to this Stack Overflow answer)
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Must(template.ParseFiles("index.tmpl"))
model := todo.NewList()
// render the template into a buffer
var buf bytes.Buffer
err := templ.Execute(&buf, model)
if err != nil {
panic(err)
}
// check that the template can be parsed as (lenient) XML
decoder := xml.NewDecoder(bytes.NewReader(buf.Bytes()))
decoder.Strict = false
decoder.AutoClose = xml.HTMLAutoClose
decoder.Entity = xml.HTMLEntity
for {
_, err := decoder.Token()
switch err {
case io.EOF:
return // We're done, it's valid!
case nil:
// do nothing
default:
t.Fatalf("Error parsing html: %s", err)
}
}
}
This code configures the HTML parser to have the right level of leniency
for HTML, and then parses the HTML token by token. Indeed, we see the error
message we wanted:
--- FAIL: Test_wellFormedHtml (0.00s) index_template_test.go:61: Error parsing html: XML syntax error on line 4: unexpected end element </p>
In Java, a versatile library to use is jsoup:
Java
@Test
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var model = new TodoList();
var html = template.execute(model);
var parser = Parser.htmlParser().setTrackErrors(10);
Jsoup.parse(html, "", parser);
assertThat(parser.getErrors()).isEmpty();
}
And we see it fail:
java.lang.AssertionError: Expecting empty but was:<[<1:13>: Unexpected EndTag token [</p>] when in state [InBody],
Success! Now if we copy over the contents of the TodoMVC
template to our index.tmpl
file, the test passes.
The test, however, is too verbose: we extract two helper functions, in
order to make the intention of the test clearer, and we get
Go
func Test_wellFormedHtml(t *testing.T) { model := todo.NewList() buf := renderTemplate("index.tmpl", model) assertWellFormedHtml(t, buf) }
Java
@Test void indexIsSoundHtml() { var model = new TodoList(); var html = renderTemplate("/index.tmpl", model); assertSoundHtml(html); }
Level 2: testing HTML structure
What else should we test?
We know that the looks of a page can only be tested, ultimately, by a
human looking at how it is rendered in a browser. However, there is often
logic in templates, and we want to be able to test that logic.
One might be tempted to test the rendered HTML with string equality,
but this technique fails in practice, because templates contain a lot of
details that make string equality assertions impractical. The assertions
become very verbose, and when reading the assertion, it becomes difficult
to understand what it is that we’re trying to prove.
What we need
is a technique to assert that some parts of the rendered HTML
correspond to what we expect, and to ignore all the details we don’t
care about. One way to do this is by running queries with the CSS selector language:
it is a powerful language that allows us to select the
elements that we care about from the whole HTML document. Once we have
selected those elements, we (1) count that the number of element returned
is what we expect, and (2) that they contain the text or other content
that we expect.
The UI that we are supposed to generate looks like this:
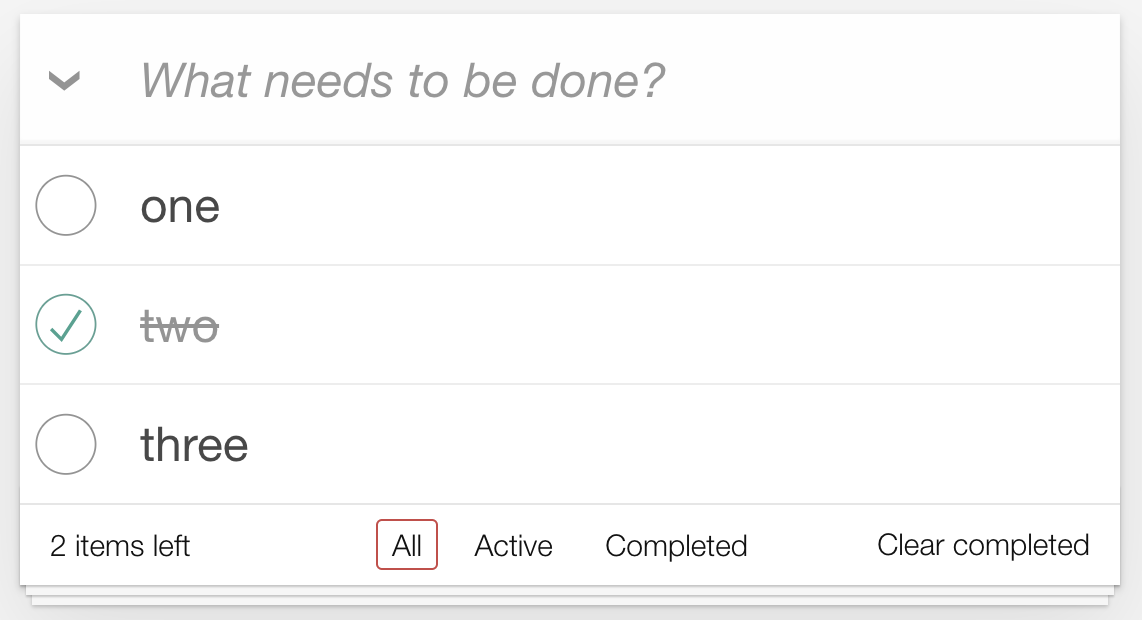
There are several details that are rendered dynamically:
- The number of items and their text content change, obviously
- The style of the todo-item changes when it’s completed (e.g., the
second) - The “2 items left” text will change with the number of non-completed
items - One of the three buttons “All”, “Active”, “Completed” will be
highlighted, depending on the current url; for instance if we decide that the
url that shows only the “Active” items is/active
, then when the current url
is/active
, the “Active” button should be surrounded by a thin red
rectangle - The “Clear completed” button should only be visible if any item is
completed
Each of this concerns can be tested with the help of CSS selectors.
This is a snippet from the TodoMVC template (slightly simplified). I
have not yet added the dynamic bits, so what we see here is static
content, provided as an example:
index.tmpl
<section class="todoapp"> <ul class="todo-list"> <!-- These are here just to show the structure of the list items --> <!-- List items should get the class `completed` when marked as completed --> <li class="completed"> ② <div class="view"> <input class="toggle" type="checkbox" checked> <label>Taste JavaScript</label> ① <button class="destroy"></button> </div> </li> <li> <div class="view"> <input class="toggle" type="checkbox"> <label>Buy a unicorn</label> ① <button class="destroy"></button> </div> </li> </ul> <footer class="footer"> <!-- This should be `0 items left` by default --> <span class="todo-count"><strong>0</strong> item left</span> ⓷ <ul class="filters"> <li> <a class="selected" href="#/">All</a> ④ </li> <li> <a href="#/active">Active</a> </li> <li> <a href="#/completed">Completed</a> </li> </ul> <!-- Hidden if no completed items are left ↓ --> <button class="clear-completed">Clear completed</button> ⑤ </footer> </section>
By looking at the static version of the template, we can deduce which
CSS selectors can be used to identify the relevant elements for the 5 dynamic
features listed above:
feature | CSS selector | |
---|---|---|
① | All the items | ul.todo-list li |
② | Completed items | ul.todo-list li.completed |
⓷ | Items left | span.todo-count |
④ | Highlighted navigation link | ul.filters a.selected |
⑤ | Clear completed button | button.clear-completed |
We can use these selectors to focus our tests on just the things we want to test.
Testing HTML content
The first test will look for all the items, and prove that the data
set up by the test is rendered correctly.
func Test_todoItemsAreShown(t *testing.T) { model := todo.NewList() model.Add("Foo") model.Add("Bar") buf := renderTemplate(model) // assert there are two <li> elements inside the <ul class="todo-list"> // assert the first <li> text is "Foo" // assert the second <li> text is "Bar" }
We need a way to query the HTML document with our CSS selector; a good
library for Go is goquery, that implements an API inspired by jQuery.
In Java, we keep using the same library we used to test for sound HTML, namely
jsoup. Our test becomes:
Go
func Test_todoItemsAreShown(t *testing.T) { model := todo.NewList() model.Add("Foo") model.Add("Bar") buf := renderTemplate("index.tmpl", model) // parse the HTML with goquery document, err := goquery.NewDocumentFromReader(bytes.NewReader(buf.Bytes())) if err != nil { // if parsing fails, we stop the test here with t.FatalF t.Fatalf("Error rendering template %s", err) } // assert there are two <li> elements inside the <ul class="todo-list"> selection := document.Find("ul.todo-list li") assert.Equal(t, 2, selection.Length()) // assert the first <li> text is "Foo" assert.Equal(t, "Foo", text(selection.Nodes[0])) // assert the second <li> text is "Bar" assert.Equal(t, "Bar", text(selection.Nodes[1])) } func text(node *html.Node) string { // A little mess due to the fact that goquery has // a .Text() method on Selection but not on html.Node sel := goquery.Selection{Nodes: []*html.Node{node}} return strings.TrimSpace(sel.Text()) }
Java
@Test void todoItemsAreShown() throws IOException { var model = new TodoList(); model.add("Foo"); model.add("Bar"); var html = renderTemplate("/index.tmpl", model); // parse the HTML with jsoup Document document = Jsoup.parse(html, ""); // assert there are two <li> elements inside the <ul class="todo-list"> var selection = document.select("ul.todo-list li"); assertThat(selection).hasSize(2); // assert the first <li> text is "Foo" assertThat(selection.get(0).text()).isEqualTo("Foo"); // assert the second <li> text is "Bar" assertThat(selection.get(1).text()).isEqualTo("Bar"); }
If we still haven’t changed the template to populate the list from the
model, this test will fail, because the static template
todo items have different text:
Go
--- FAIL: Test_todoItemsAreShown (0.00s) index_template_test.go:44: First list item: want Foo, got Taste JavaScript index_template_test.go:49: Second list item: want Bar, got Buy a unicorn
Java
IndexTemplateTest > todoItemsAreShown() FAILED org.opentest4j.AssertionFailedError: Expecting: <"Taste JavaScript"> to be equal to: <"Foo"> but was not.
We fix it by making the template use the model data:
Go
<ul class="todo-list"> {{ range .Items }} <li> <div class="view"> <input class="toggle" type="checkbox"> <label>{{ .Title }}</label> <button class="destroy"></button> </div> </li> {{ end }} </ul>
Java – jmustache
<ul class="todo-list"> {{ #allItems }} <li> <div class="view"> <input class="toggle" type="checkbox"> <label>{{ title }}</label> <button class="destroy"></button> </div> </li> {{ /allItems }} </ul>
Test both content and soundness at the same time
Our test works, but it is a bit verbose, especially the Go version. If we’re going to have more
tests, they will become repetitive and difficult to read, so we make it more concise by extracting a helper function for parsing the html. We also remove the
comments, as the code should be clear enough
Go
func Test_todoItemsAreShown(t *testing.T) { model := todo.NewList() model.Add("Foo") model.Add("Bar") buf := renderTemplate("index.tmpl", model) document := parseHtml(t, buf) selection := document.Find("ul.todo-list li") assert.Equal(t, 2, selection.Length()) assert.Equal(t, "Foo", text(selection.Nodes[0])) assert.Equal(t, "Bar", text(selection.Nodes[1])) } func parseHtml(t *testing.T, buf bytes.Buffer) *goquery.Document { document, err := goquery.NewDocumentFromReader(bytes.NewReader(buf.Bytes())) if err != nil { // if parsing fails, we stop the test here with t.FatalF t.Fatalf("Error rendering template %s", err) } return document }
Java
@Test void todoItemsAreShown() throws IOException { var model = new TodoList(); model.add("Foo"); model.add("Bar"); var html = renderTemplate("/index.tmpl", model); var document = parseHtml(html); var selection = document.select("ul.todo-list li"); assertThat(selection).hasSize(2); assertThat(selection.get(0).text()).isEqualTo("Foo"); assertThat(selection.get(1).text()).isEqualTo("Bar"); } private static Document parseHtml(String html) { return Jsoup.parse(html, ""); }
Much better! At least in my opinion. Now that we extracted the parseHtml
helper, it’s
a good idea to check for sound HTML in the helper:
Go
func parseHtml(t *testing.T, buf bytes.Buffer) *goquery.Document {
assertWellFormedHtml(t, buf)
document, err := goquery.NewDocumentFromReader(bytes.NewReader(buf.Bytes()))
if err != nil {
// if parsing fails, we stop the test here with t.FatalF
t.Fatalf("Error rendering template %s", err)
}
return document
}
Java
private static Document parseHtml(String html) { var parser = Parser.htmlParser().setTrackErrors(10); var document = Jsoup.parse(html, "", parser); assertThat(parser.getErrors()).isEmpty(); return document; }
And with this, we can get rid of the first test that we wrote, as we are now testing for sound HTML all the time.
The second test
Now we are in a good position for testing more rendering logic. The
second dynamic feature in our list is “List items should get the class
completed
when marked as completed”. We can write a test for this:
Go
func Test_completedItemsGetCompletedClass(t *testing.T) { model := todo.NewList() model.Add("Foo") model.AddCompleted("Bar") buf := renderTemplate("index.tmpl", model) document := parseHtml(t, buf) selection := document.Find("ul.todo-list li.completed") assert.Equal(t, 1, selection.Size()) assert.Equal(t, "Bar", text(selection.Nodes[0])) }
Java
@Test void completedItemsGetCompletedClass() { var model = new TodoList(); model.add("Foo"); model.addCompleted("Bar"); var html = renderTemplate("/index.tmpl", model); Document document = Jsoup.parse(html, ""); var selection = document.select("ul.todo-list li.completed"); assertThat(selection).hasSize(1); assertThat(selection.text()).isEqualTo("Bar"); }
And this test can be made green by adding this bit of logic to the
template:
Go
<ul class="todo-list">
{{ range .Items }}
<li class="{{ if .IsCompleted }}completed{{ end }}">
<div class="view">
<input class="toggle" type="checkbox">
<label>{{ .Title }}</label>
<button class="destroy"></button>
</div>
</li>
{{ end }}
</ul>
Java – jmustache
<ul class="todo-list">
{{ #allItems }}
<li class="{{ #isCompleted }}completed{{ /isCompleted }}">
<div class="view">
<input class="toggle" type="checkbox">
<label>{{ title }}</label>
<button class="destroy"></button>
</div>
</li>
{{ /allItems }}
</ul>
So little by little, we can test and add the various dynamic features
that our template should have.
Contact Softscribble for your software Requirement
Contact Now